Byte-sized functional programming: Filter first
Byte-sized functional programming: Filter first
Often when working with a list, we only want to work with a subset of a list that meets some criteria. All non-zero values, for example, or all users that have a given role. The procedural way to do that is to stick an if
statement inside a foreach
loop:
<?php
foreach ($list as $value) {
If (!meets_criteria($value)) {
continue;
}
// ...
}
?>
That mixes up the filtering with the iteration, though. It also doesn't work if we're using `array_map()`.
Instead, we can make stripping down the list a separate operation called "filter." PHP offers the array_filter()
function for that purpose.
<?php
$criteria = fn(User $user): bool => $user->hasRole('moderator');
$filtered = array_filter($users, $criteria);
?>
Now we can work with the `$filtered` list, which has only the values we want. That could be a simple foreach
loop, or, better, it's now ideally suited for use with
array_map()
.
Want to know more about functional programming and PHP? Read the whole book on the topic: Thinking Functionally in PHP.
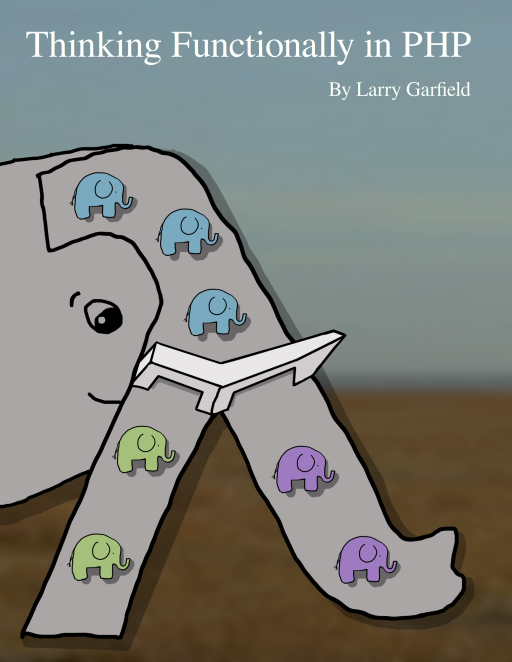